CRUD web apps deal with structured data, like rows in database tables. We'll be using JS objects for that.
An object is a collection of data and functions. You've already been making objects, without knowing it. You know those namespacing things?
<script>
"use strict";
var CourseScore = CourseScore || {};
CourseScore
is a JS object.
JSON
Suppose we want a catalog of university courses. Let's start with one course for now. Here's how you could store information about it:
var course = {
id: 42,
title: "Web apps for happy dogs",
maxEnrollment: 40,
location: "Monty Hall 301"
};
This is JSON, which stands for JavaScript object notation. An object is a collection of properties, each one with a name and a value.
JSON is a standard format for servers and clients to communicate with each other. We're sticking to browsers only, but still using JSON.
Accessing JSON properties
This would show the title of the course:
alert("Course title: " + course.title);
The object name, a dot, then the property.
Records and JS objects
When people talk about CRUD apps, they often use words that apply to relational databases. You've probably learned about relational databases already.
Here's a database table, called zombiedata
:
It's rows, and columns. Each row is also called a record. Each column is also called a field.
One of the fields is called the primary key. Its value uniquely identifies each record. z_id
is the PK in this table.
You can use a query to fetch some records from a table. The group of records fetched by the query is called a record set. For example, this SQL SELECT query will return a record set with four records:
SELECT * FROM zombiedata WHERE dept = 6;
Since you already know these concepts, let's use them in this course.
This is really a JS object:
var course = {
id: 42,
title: "Web apps for happy dogs",
maxEnrollment: 40,
location: "Monty Hall 301"
};
But we'll call it a record as well, since in a CRUD app, it looks and acts like a record.
We'll call the properties (like title
, and maxEnrollment
) fields, since they look and act like fields.
We'll call the id
field the primary key, since it looks and acts like primary key.
In fact, JS objects are more flexible than database records. One difference is that properties can be functions. That's why our namespace objects can have functions, like this:
var CourseScore = CourseScore || {};
CourseScore.showScore = function() {
So, in the rest of the course, we'll use words like field, primary key, record, and record set, to take advantage of what you already know.
Showing a course
Here's a simple page showing one course. It starts like this:
Click the button to see the course's details:
Here's the HTML:
<h1>Course Catalog</h1>
<p>Click the button to see details for a course.</p>
<p>
<button onclick="CourseCatalog.showCourse()"
type="button" class="btn btn-primary"
title="Show deets">Course deets</button>
</p>
<ul id="courseDeets" class="hide-on-load">
<li>Title: <span id="courseTitle"></span></li>
<li>Maximum enrollment: <span id="courseMaxEnrollment"></span></li>
<li>Location: <span id="courseLocation"></span></li>
</ul>
Recall that hide-on-load
is defined like this:
.hide-on-load {
display: none;
}
So, when the page loads, the output container, courseDeets
, is hidden.
Here's the JS:
CourseCatalog.showCourse = function() {
$("#courseTitle").html(course.title);
$("#courseMaxEnrollment").html(course.maxEnrollment);
$("#courseLocation").html(course.location);
$("#courseDeets").show('slow');
};
The code fills in the <span>
tags with data from the course
object, then shows the container.
There is something new on the last line:
$("#courseDeets").show('slow');
The argument 'slow'
creates an animated effect.
Another way
Here's another way to do things. First, the HTML.
We've replaced this…
<ul id="courseDeets" class="hide-on-load">
<li>Title: <span id="courseTitle"></span></li>
<li>Maximum enrollment: <span id="courseMaxEnrollment"></span></li>
<li>Location: <span id="courseLocation"></span></li>
</ul>
… with…
<div id="courseContainer" class="hide-on-load"></div>
The container is now an empty <div>
. Here's the JS:
var outputHtml =
"<ul>" +
" <li>Title: " + course.title + "</li>" +
" <li>Maximum enrollment: " + course.maxEnrollment + "</li>" +
" <li>Location: " + course.location + "</li>" +
"</ul>";
$("#courseContainer")
.html(outputHtml)
.show('slow');
This time, the JS builds the HTML in a variable. Then it injects the HTML into the page.
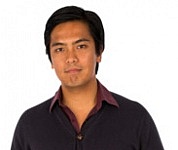
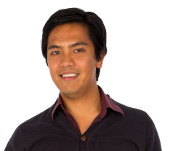
Usually, the ids are just numbers, with no meaning. Users don't care what the ids are. Whether a course has an id of 42, 89, 1738, or 4744734 doesn't matter to them, as long as the computer keeps the records straight. That's why our JS didn't show the key.
You'll learn now ids are used later.
Exercise
(If you were logged in as a student, you could submit an exercise solution, and get some feedback.)
Summary
- A JSON object is like a row in a database table.
- JS code can show an object by (1) inserting values into existing HTML, or (2) creating new HTML that includes the values.
show('slow')
uses animation.