The story so far
In the last lesson, we created an app that shows a university course catalog. Now, we're going to writing an app about a pack of dogs.
Courses and dogs have much in common.
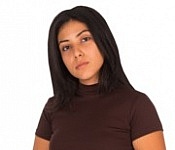
Courses, and dogs. You mean the animals, right?
OK, courses and dogs don't have much in common, but their data and apps do.
Records
Start with the data. Here's a course record:
{
id: 42,
title: "Web apps for happy dogs",
maxEnrollment: 40,
location: "Monty Hall 301"
}
Here's a dog record:
{
id: 11,
name: "Bufford",
description: "She can sniff it out.",
weight: 26
}
A record is data about one entity: a course, or a dog. Each record has fields, aka properties.
One of those fields is an id that uniquely identifies the record. Only one course has an id of 42. Only one dog has an id of 11. The field is called the primary key.
Courses and dogs are different, but the data about them is in records, with fields, one of which is a key.
Record sets
Here's part of a course record set.
var catalog = [
{
id: 42,
title: "Web apps for happy dogs",
maxEnrollment: 40,
location: "Monty Hall 301"
},
{
id: 155,
title: "Databases for happy dogs",
maxEnrollment: 32,
location: "Monty Hall 301"
},
...
];
Now for dogs:
var dogPack = [
{
id: 11,
name: "Bufford",
description: "She can sniff it out.",
weight: 26
},
{
id: 24,
name: "Wold",
description: "The more relaxed dog in the pack.",
weight: 17
},
...
];
Data for a collection of courses – a catalog – is kept in a record set.
Data for a collection of dogs – a pack – is kept in a record set.
Interfaces
Here's an interface for looking at a course catalog:
Here's an interface for looking at a dog pack:
The interfaces aren't exactly the same, but they're more similar than different.
Deep structure
As people become more skilled, they see beyond the surface of a task, into the deep structure. They know that while courses, dogs, products, and employees are all different things, the data that describes them is organized in the same way.
The code is similar as well. Here's some pseudocode for showing a record set, like the two previous screen shots:
For each record in a record set:
Make HTML for the record
Add the record's HTML to the overall record set's HTML
Put the record set's HTML into the page
This works for courses, dogs, products, employees, toys, books… thousands of use cases.
Business people see different apps, with different data, about different things. Programmers see similar data structures, and similar code.
Skilled programmers reuse code. They copy code from a course catalog app, to a dog pack app, then change it. The variable names will be different, and the table's column headings will be different, but underneath, the code is the same.
The term "deep structure" doesn't have a specific technical meaning. It's just the idea that systems that are different on the surface, can be similar underneath.
"Deep structure" is actually a term from psychology, not IT. Researchers have studied differences between novices and experts. They've found that novices often get hung up in surface details, like, "Dogs are animals, they like to sniff each others' butts, is that part of the app?" Experts will see the deep structure, and start working with records and record sets.
Patterns
A pattern is a common way of doing things. For example, the pseudocode for showing a record set is a pattern:
For each record in a record set:
Make HTML for the record
Add the record's HTML to the overall record set's HTML
Put the record set's HTML into the page
The pattern is reusable, that is, can be used in many situations. That's because the pattern is about deep structure. Check out the words it uses. Neither "course" nor "dog," but "record." Neither "course catalog" nor "dog pack," but "record set."
You've already seen some patterns. You'll see more throughout the course. They show up as quick summaries, with a link for more deets. For example:
The pattern catalog
Patterns are explained throughout the lessons. However, when you're doing a task, it's a pain to look through all the lessons until you find the pattern you need. That's what the pattern catalog is for.
The pattern catalog lists all of the patterns. For example:
The Situation column shows what the pattern helps you do. The Actions column is a quick summary of the pattern. Click on the pattern's name for the details, including code you can copy and paste.
When you're working on a task, the pattern catalog can be handy. There's a link to it in the Tools menu on every page.
Summary
- Many apps have the same deep structure.
- A pattern is a useful way of doing things.
- The pattern catalog lists patterns you can use.
- There's a link to the pattern catalog on every page.